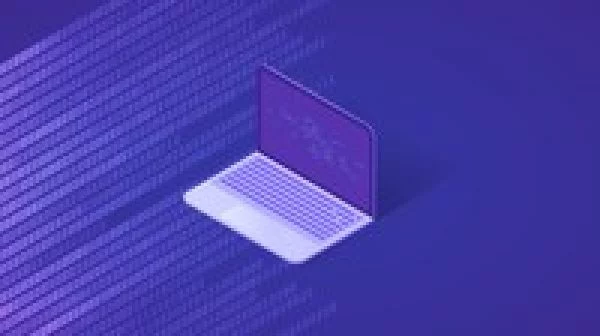
This course is for those who are interested in computer science and want to implement the algorithms and given data structures in C++ from scratch. In every chapter you will learn about the theory of a given data structure or algorithm and then you will implement them from scratch.
Chapter 1: Recursion
theory behind recursion (recursive function calls)
stack memory and heap memory
recursion and stack memory of the OS
recursive problems such as the Towers of Hanoi problem
Chapter 2: Backtracking
what is backtracking
how to solve problems with backtracking
N–queens problem
coloring problem
knight’s tour
Chapter 3: Dynamic Programming
overlapping subproblems and dynamic programming
what is memoization and abulation ?
Fibonacci numbers
knapsack problem
Chapter 4: Data Structures
data structures and abstract data types (ADTs)
arrays
linked lists
stacks
queues
binary search trees
priority queues (heaps)
associative arrays (hash tables)
Chapter 5: Graphs
directed and undirected graphs
graph traversal: breadth–first search and depth–first search
shortest path algorithms
Dijkstra’s algorithm
Bellman–Ford algorithm
Chapter 6: Substring Search Algorithms
the most relevant substring search algorithms
naive substring search
Knuth–Morris–Pratt (KMP) substring search algorithm
Rabin–Karp algorithm
Z algorithm (linear pattern matching)
Chapter 7: Sorting
stable sorting and adaptive sorting
comparison based and non–comparison based sorting algorithms
Instructor Details
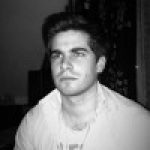
Courses : 24
Specification: Algorithms (Data Structures) Bootcamp in C++
|
14 reviews for Algorithms (Data Structures) Bootcamp in C++
Add a review Cancel reply
This site uses Akismet to reduce spam. Learn how your comment data is processed.
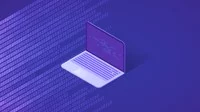
Price | $15.99 |
---|---|
Provider | |
Duration | 17.5 hours |
Year | 2022 |
Level | All |
Language | English |
Certificate | Yes |
Quizzes | Yes |

$19.99 $15.99
Thomas Zoller –
The course is good for total beginners or as a quick refresher for everyone with at least a little CS background. Author explains all topics in an easy to follow way. Examples are very abstract which is sufficient to explain the algorithms but gives no idea about practical application. There’s nothing C++ specific except that the examples are implemented in C++. So, the learner should more expect lectures about algorithms than about C++. For anyone fluent in English, watch at 1.5x times speed.
Vikram –
Instructor simply reads from the slides for most slides. Some slides have errors on them and some present less than optimal solutions for the problems posed (ex: coloring problem). There are no exercises and coding challenges to reinforce your understanding. Course Q&A is not active. So if you get stuck somewhere, you’re pretty much on your own. But the content (topics covered) is good. And the code he provides is well thought out.
Mohammad Aashik Khan –
Instructor is explaining everything related to the topic in depth. Hats off!
Lewis Bawden –
As someone with a background in C++ from experimental physics, but without a computer science background, I enjoyed this course and learnt a lot from it. It was a great introduction into basic abstract data types and basic graph search and recursive algorithms. The instructor covered interesting problems in a very clear way and slowly built up to the highest complexity problem, but without feeling like it was going at a painfully slow or lightning fast pace.
Anonymized User –
exceptional, the code runs, a very good instructor
Vinayak Kukreja –
The course is really nice and it is for everyone who needs a refresher or even a start with algorithms and data structures in C++. The pace of the course is very good, and the instructor’s knowledge regarding the subject is also very good.
Premchand Jodukallu –
high level theory (very good explanation) which we can gain by any good text book . practical ascpectsmsample programe required
Marina Landisberg –
Good explanations, I like course of this instructor
Nahim El Atmani –
I found the course a bit too boring, examples are already implemented and leave no space for tinkering and trying on our own. All those information are easily searchable online for free and would take far less time to go through than 7.5 hours.
Neil Chokriwala –
His code is really clean!!! You can learn just from the code itself and just his syntax and spacing, how he lays out comments, he picks a bunch of challenging problems. This is a really good course.
Abinash Mishra –
Author could have given some detailed and deep down view of some topic than this course will be considered as Exceptional for me.
Ankitaben Patel –
Very few courses actually in depth fundamentals of DS. These course’s not only coverage is good, it also has lots of other information and different implementation. I was afraid of addressing knapsack problem and N queen problem till now. Explanation given here made it so easy to understand. Must have course for every C++ developer.
Shubham Gupta –
expecting some more visual representations
Dan Joshua Santiva ez Gutarra –
I love Udemy